Vulkan® Memory Allocator
The industry-leading, open source, memory allocation library for the Vulkan API.
The Vulkan® Memory Allocator (VMA) library provides a simple and easy to integrate API to help you allocate memory for Vulkan® buffer and image storage.
Download the latest version - v3.2.1
- Fixed an assert in
vmaCreateAllocator
function incorrectly failing when Vulkan version 1.4 is used. - Fix for importing function
vkGetPhysicalDeviceMemoryProperties2
/vkGetPhysicalDeviceMemoryProperties2KHR
whenVMA_DYNAMIC_VULKAN_FUNCTIONS
macro is enabled. - Other minor fixes and improvements.
Benefits
The library is battle-ready, and integrated into the majority of Vulkan® game titles on PC, as well as the Google Filament rendering engine, the official Khronos® Group Vulkan® Samples, and many other open source projects for Android™, Linux, MacOS, and Windows®.
This library can help game developers to manage memory allocations and resource creation by offering some higher-level functions:
- Functions that help to choose correct and optimal memory type based on intended usage of the memory.
- Required or preferred traits of the memory are expressed using higher-level description comparing to Vulkan flags.
- Functions that allocate memory blocks, reserve and return parts of them (
VkDeviceMemory
+ offset + size) to the user.- Library keeps track of allocated memory blocks, used and unused ranges inside them, finds best matching unused ranges for new allocations, respects all the rules of alignment and buffer/image granularity.
- Functions that can create an image/buffer, allocate memory for it and bind them together – all in one call.
Additional features
- Self-contained C++ library in single header file. No external dependencies other than the standard C and C++ library and Vulkan.
- Public interface in C, in same convention as Vulkan API. Implementation in C++.
- Platform-independent, but developed and tested on Windows using Visual Studio. Continuous integration setup for Windows and Linux. Used also on Android, MacOS, and other platforms.
- Support for memory mapping, reference-counted internally.
- Support for persistently mapped memory: Just allocate with appropriate flag and access the pointer to already mapped memory.
- Linear allocator: Create a pool with linear algorithm and use it for much faster allocations and deallocations in free-at-once, stack, double stack, or ring buffer fashion.
- Defragmentation of GPU and CPU memory: Let the library move data around to free some memory blocks and make your allocations better compacted.
- Statistics: Obtain brief or detailed statistics about the amount of memory used, unused, number of allocated blocks, number of allocations etc. - globally, per memory heap, and per memory type.
- Debug annotations: Associate custom void* pUserData and debug char* pName with each allocation.
- JSON dump: Output a JSON string with detailed map of internal state, including allocations, their string names, and gaps between them.
- Convert JSON dump into a picture to visualize your memory.
- Support for interoperability with OpenGL.
- Virtual allocator: Interface for using core allocation algorithm to allocate any custom data, e.g. pieces of one large buffer.
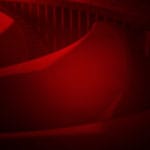
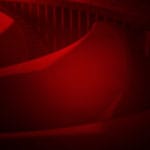
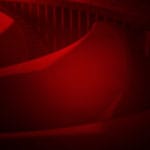
Version history
- Fixed an assert in
vmaCreateAllocator
function incorrectly failing when Vulkan version 1.4 is used. - Fix for importing function
vkGetPhysicalDeviceMemoryProperties2
/vkGetPhysicalDeviceMemoryProperties2KHR
whenVMA_DYNAMIC_VULKAN_FUNCTIONS
macro is enabled. - Other minor fixes and improvements.
This release adds the following features:
- Additions to the library API:
- Added support for Vulkan 1.4.
- Added support for
VK_KHR_external_memory_win32
extension –VMA_ALLOCATOR_CREATE_KHR_EXTERNAL_MEMORY_WIN32_BIT
flag,vmaGetMemoryWin32Handle
function, and a whole new documentation chapter about it.
- Other changes:
- Fixed thread safety issue.
- Many other bug fixes and improvements in the library code, documentation, sample app, Cmake script, mostly to improve compatibility with various compilers and GPUs.
This release adds the following features:
- Added convenience functions
vmaCopyMemoryToAllocation
,vmaCopyAllocationToMemory
. - Added functions
vmaCreateAliasingBuffer2
,vmaCreateAliasingImage2
that offer creating a buffer/image in an existing allocation with additionalallocationLocalOffset
. - Added function
vmaGetAllocationInfo2
, structureVmaAllocationInfo2
that return additional information about an allocation, useful for interop with other APIs. - Added callback
VmaDefragmentationInfo::pfnBreakCallback
that allows breaking long execution ofvmaBeginDefragmentation
. Also addedPFN_vmaCheckDefragmentationBreakFunction
,VmaDefragmentationInfo::pBreakCallbackUserData
. - Added support for VK_KHR_maintenance4 extension –
VMA_ALLOCATOR_CREATE_KHR_MAINTENANCE4_BIT
flag. - Added support for VK_KHR_maintenance5 extension –
VMA_ALLOCATOR_CREATE_KHR_MAINTENANCE5_BIT
flag. - Changes in debug and configuration macros:
- Split macros into separate
VMA_DEBUG_LOG
andVMA_DEBUG_LOG_FORMAT
. - Added macros
VMA_ASSERT_LEAK
,VMA_LEAK_LOG_FORMAT
separate from normalVMA_ASSERT
,VMA_DEBUG_LOG_FORMAT
. - Added macro
VMA_EXTENDS_VK_STRUCT
.
- Split macros into separate
- Countless bug fixes and improvements in the code and documentation, mostly to improve compatibility with various compilers and GPUs, including:
- Fixed missing
#include
that resulted in compilation error aboutsnprintf
not declared on some compilers. - Fixed main memory type selection algorithm for GPUs that have no
HOST_CACHED
memory type, like Raspberry Pi.
- Fixed missing
- Major changes in Cmake script.
- Fixes in GpuMemDumpVis.py script.
- Fixes in defragmentation algorithm.
- Fixes in GpuMemDumpVis.py regarding image height calculation.
- Other bug fixes, optimizations, and improvements in the code and documentation.
- Added new API for selecting preferred memory type.
- Added new defragmentation API and algorithm, replacing the old one.
- Redesigned API for statistics, replacing the old one.
- Added “Virtual allocator” feature – possibility to use core allocation algorithms for allocation of custom memory, not necessarily Vulkan device memory.
-
VmaAllocation
now keeps bothvoid* pUserData
andchar* pName
. Added functionvmaSetAllocationName
, memberVmaAllocationInfo::pName
. - Clarified and cleaned up various ways of importing Vulkan functions. Added members
VmaVulkanFunctions::vkGetInstanceProcAddr
, andvkGetDeviceProcAddr
, which are now required when usingVMA_DYNAMIC_VULKAN_FUNCTIONS
.
- Added support for Vulkan 1.1.
- Added member
VmaAllocatorCreateInfo::vulkanApiVersion
. - When Vulkan 1.1 is used, there is no need to enable
VK_KHR_dedicated_allocation
orVK_KHR_bind_memory2
extensions, as they are promoted to Vulkan itself.
- Added member
- Added support for query for memory budget and staying within the budget.
- Added function
vmaGetBudget
, structureVmaBudget
. This can also serve as simple statistics, more efficient thanvmaCalculateStats
. - By default the budget it is estimated based on memory heap sizes. It may be queried from the system using
VK_EXT_memory_budget
extension if you useVMA_ALLOCATOR_CREATE_EXT_MEMORY_BUDGET_BIT
flag andVmaAllocatorCreateInfo::instance
member. - Added flag
VMA_ALLOCATION_CREATE_WITHIN_BUDGET_BIT
that fails an allocation if it would exceed the budget.
- Added function
- Added new memory usage options:
-
VMA_MEMORY_USAGE_CPU_COPY
for memory that is preferably notDEVICE_LOCAL
but not guaranteed to beHOST_VISIBLE
. -
VMA_MEMORY_USAGE_GPU_LAZILY_ALLOCATED
for memory that isLAZILY_ALLOCATED
.
-
- Added support for
VK_KHR_bind_memory2
extension:- Added
VMA_ALLOCATION_CREATE_DONT_BIND_BIT
flag that lets you create both buffer/image and allocation, but don’t bind them together. - Added flag
VMA_ALLOCATOR_CREATE_KHR_BIND_MEMORY2_BIT
, functionsvmaBindBufferMemory2
,vmaBindImageMemory2
that let you specify additional local offset andpNext
pointer while binding.
- Added
- Added functions
vmaSetPoolName
,vmaGetPoolName
that let you assign string names to custom pools. JSON dump file format and VmaDumpVis tool is updated to show these names. - Defragmentation is legal only on buffers and images in
VK_IMAGE_TILING_LINEAR
. This is due to the way it is currently implemented in the library and the restrictions of the Vulkan specification. Clarified documentation in this regard.
- New, more powerful defragmentation:
- Added structure
VmaDefragmentationInfo2
, functionsvmaDefragmentationBegin
,vmaDefragmentationEnd
. - Added support for defragmentation of GPU memory.
- Defragmentation of CPU memory now uses
memmove
, so it can move data to overlapping regions. - Defragmentation of CPU memory is now available for memory types that are
HOST_VISIBLE
but notHOST_COHERENT
. - Added structure member
VmaVulkanFunctions::vkCmdCopyBuffer
. - Major internal changes in defragmentation algorithm.
- VmaReplay: added parameters:
--DefragmentAfterLine
,--DefragmentationFlags
. - Old interface (structure
VmaDefragmentationInfo
, functionvmaDefragment
) is now deprecated.
- Added structure
- Added buddy algorithm, available for custom pools – flag
VMA_POOL_CREATE_BUDDY_ALGORITHM_BIT
. - Added convenience functions for multiple allocations and deallocations at once, intended for sparse binding resources – functions
vmaAllocateMemoryPages
,vmaFreeMemoryPages
. - Added function that tries to resize existing allocation in place:
vmaResizeAllocation
. - Added flags for allocation strategy:
VMA_ALLOCATION_CREATE_STRATEGY_BEST_FIT_BIT
,VMA_ALLOCATION_CREATE_STRATEGY_WORST_FIT_BIT
,VMA_ALLOCATION_CREATE_STRATEGY_FIRST_FIT_BIT
, and their aliases:VMA_ALLOCATION_CREATE_STRATEGY_MIN_MEMORY_BIT
,VMA_ALLOCATION_CREATE_STRATEGY_MIN_TIME_BIT
,VMA_ALLOCATION_CREATE_STRATEGY_MIN_FRAGMENTATION_BIT
.
- Added linear allocation algorithm, accessible for custom pools, that can be used as free-at-once, stack, double stack, or ring buffer. See “Linear allocation algorithm” documentation chapter.
- Added
VMA_POOL_CREATE_LINEAR_ALGORITHM_BIT
,VMA_ALLOCATION_CREATE_UPPER_ADDRESS_BIT
.
- Added
- Added feature to record sequence of calls to the library to a file and replay it using dedicated application. See documentation chapter “Record and replay”.
- Recording: added
VmaAllocatorCreateInfo::pRecordSettings
. - Replaying: added VmaReplay project.
- Recording file format: added document “docs/Recording file format.md”.
- Recording: added
- Improved support for non-coherent memory.
- Added functions:
vmaFlushAllocation
,vmaInvalidateAllocation
. nonCoherentAtomSize
is now respected automatically.- Added
VmaVulkanFunctions::vkFlushMappedMemoryRanges
,vkInvalidateMappedMemoryRanges
.
- Added functions:
- Improved debug features related to detecting incorrect mapped memory usage. See documentation chapter “Debugging incorrect memory usage”.
- Added debug macro
VMA_DEBUG_DETECT_CORRUPTION
, functionsvmaCheckCorruption
,vmaCheckPoolCorruption
. - Added debug macro
VMA_DEBUG_INITIALIZE_ALLOCATIONS
to initialize contents of allocations with a bit pattern. - Changed behavior of
VMA_DEBUG_MARGIN
macro – it now adds margin also before first and after last allocation in a block.
- Added debug macro
- Changed format of JSON dump returned by
vmaBuildStatsString
(not backward compatible!).- Custom pools and memory blocks now have IDs that don’t change after sorting.
- Added properties: “CreationFrameIndex”, “LastUseFrameIndex”, “Usage”.
- Changed VmaDumpVis tool to use these new properties for better coloring.
- Changed behavior of
vmaGetAllocationInfo
andvmaTouchAllocation
to updateallocation.lastUseFrameIndex
even if allocation cannot become lost.
- Introduction of
VmaAllocation
handle that you must retrieve from allocation functions and pass to deallocation functions next to normalVkBuffer
andVkImage
. - Introduction of
VmaAllocationInfo
structure that you can retrieve fromVmaAllocation
handle to access parameters of the allocation (likeVkDeviceMemory
and offset) instead of retrieving them directly from allocation functions. - Support for reference-counted mapping and persistently mapped allocations – see
vmaMapMemory
,VMA_ALLOCATION_CREATE_MAPPED_BIT
. - Support for custom memory pools – see
VmaPool
handle,VmaPoolCreateInfo
structure,vmaCreatePool
function. - Support for defragmentation (compaction) of allocations – see function
vmaDefragment
and related structures. - Support for “lost allocations” – see appropriate chapter on documentation Main Page.
- Initial release.
Related to Vulkan® Memory Allocator
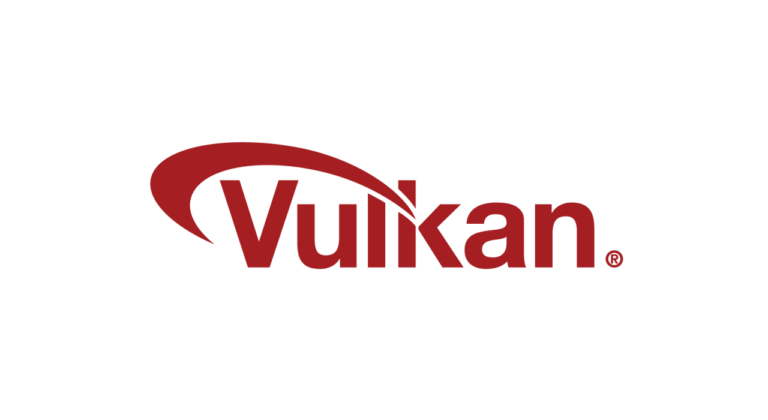
Vulkan Memory Allocator v3.1, with many fixes and improvements, is out now!
VMA V3.1 gathers fixes and improvements, mostly GitHub issues/PRs, including improved compatibility with various compilers and GPUs.

Memory Management in the APEX Engine – Digital Dragons 2022
This talk is a joint-presentation with Avalanche Studios Group explaining how their in-house APEX Engine manages memory with the help of VMA/D3D12MA.
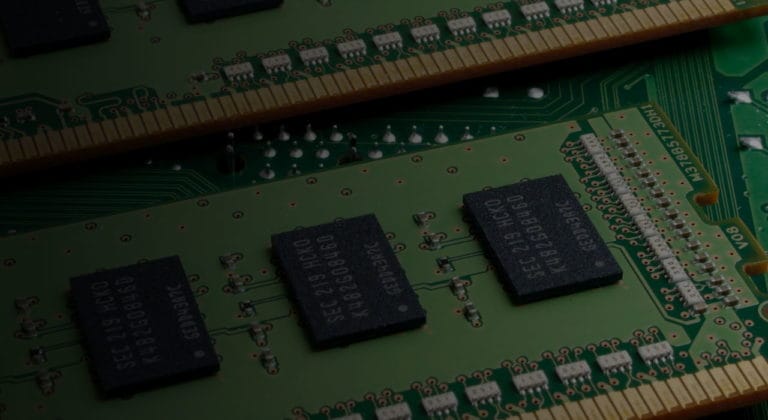
The latest Vulkan SDK now ships with Vulkan Memory Allocator (VMA)
VMA appears as an optional component that can be selected in the Vulkan SDK 1.3.216.0 installer.
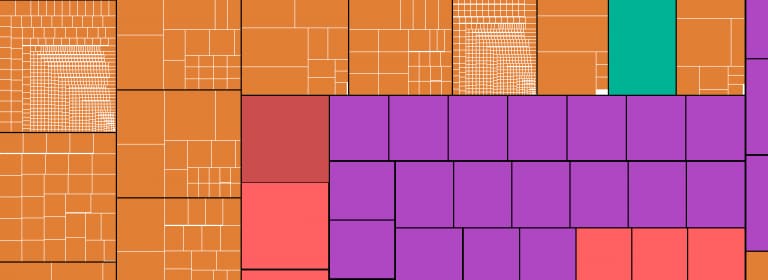
Introducing Radeon™ Memory Visualizer
Appreciate your allocations. Obliterate your oversubscription. And make good those memory leaks. Show your video memory some love, with Radeon™ Memory Visualizer.
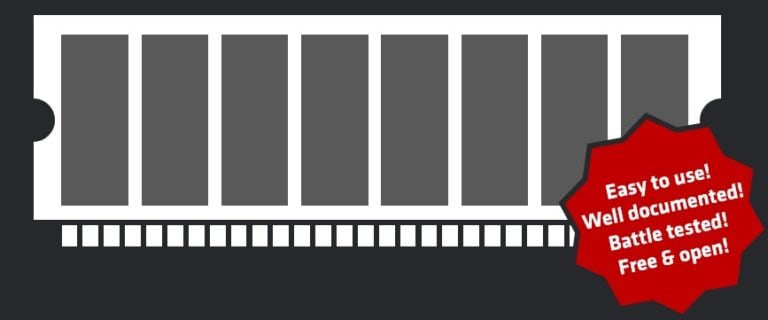
Vulkan Memory Allocator 2.3.0
The latest Vulkan Memory Allocator v2.3.0 adds support for Vulkan 1.1, support for query for memory budget, and support for VK_KHR_bind_memory2 extension.
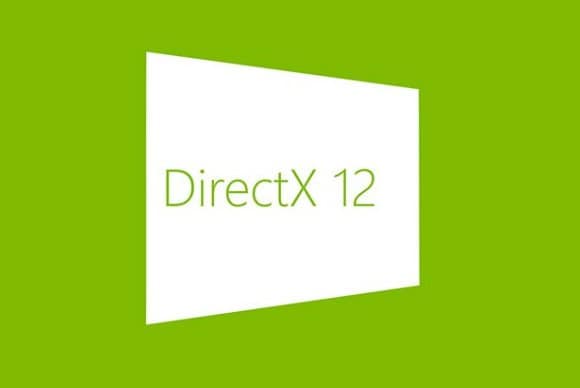
D3D12 Memory Allocator 1.0.0
Announcing the D3D12 version of our Memory Allocator tool.
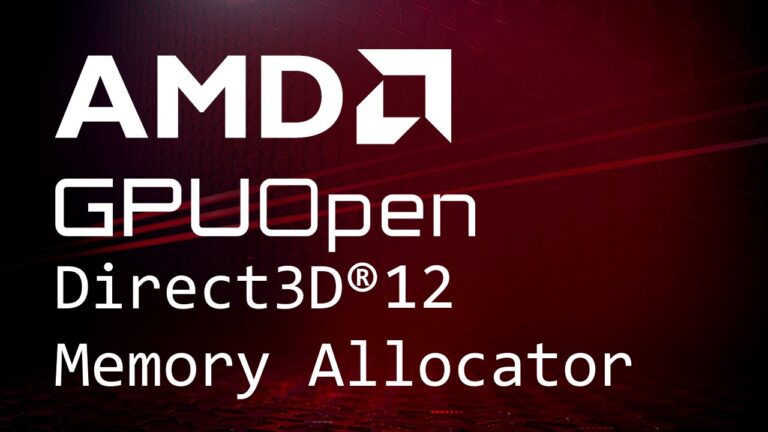
D3D12 Memory Allocator
The D3D12 Memory Allocator (D3D12MA) is a C++ library that provides a simple and easy-to-integrate API to help you allocate memory for DirectX®12 buffers and textures.
Our other SDKs
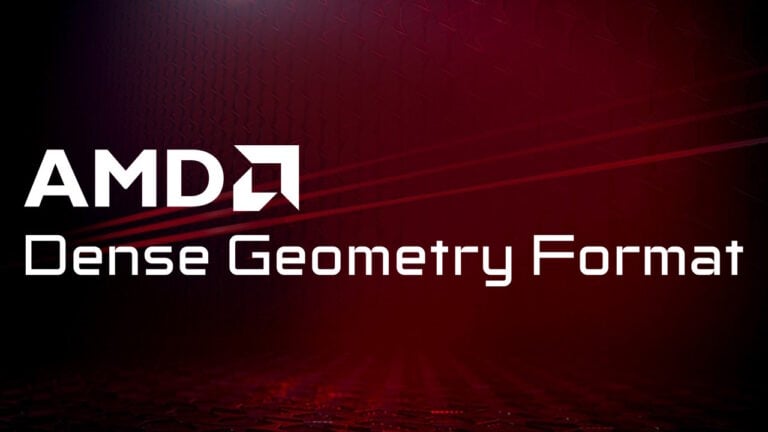
Dense Geometry Compression Format (DGF) is our block-based geometry compression technology. It is a hardware-friendly format, supported by future GPU architectures.
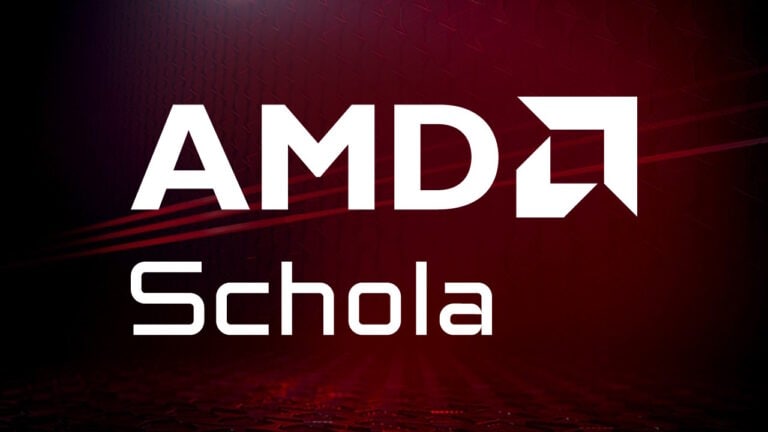
AMD Schola is a library for developing reinforcement learning (RL) agents in Unreal Engine and training with your favorite python-based RL Frameworks.
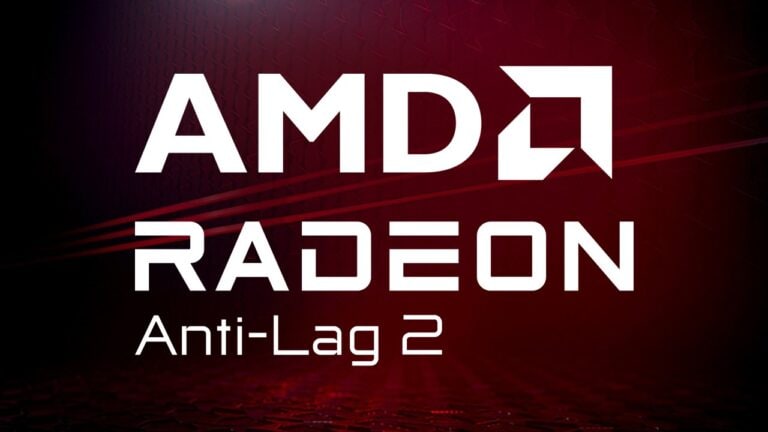
AMD Radeon™ Anti-Lag 2 reduces the system latency by applying frame alignment between the CPU and GPU jobs.
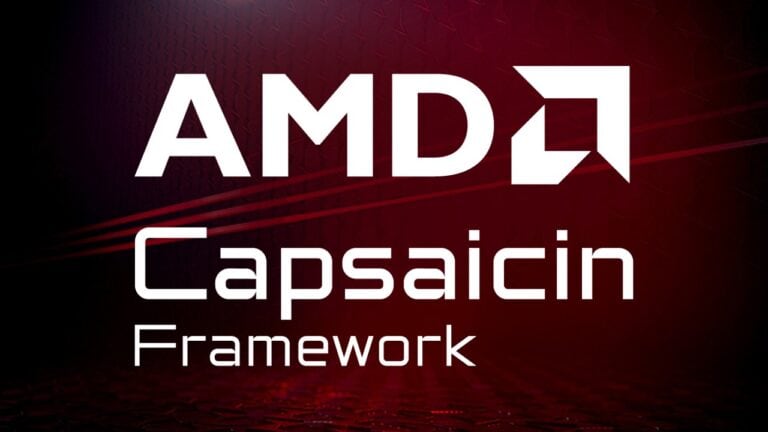
Capsaicin is a Direct3D12 framework for real-time graphics research which implements the GI-1.0 technique and a reference path-tracer.
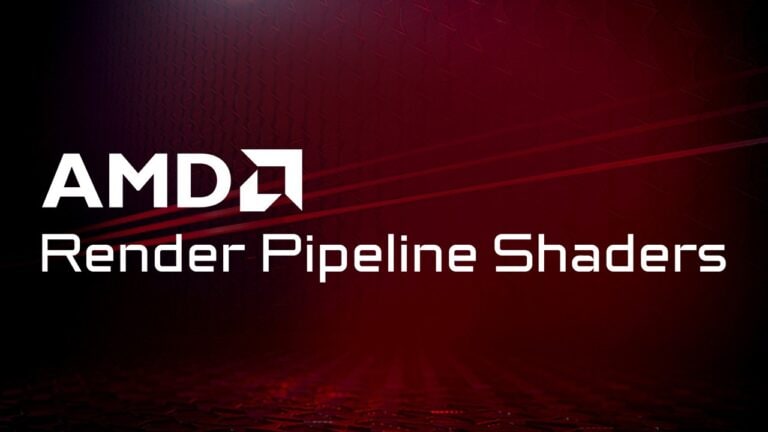
The Render Pipeline Shaders (RPS) SDK provides a framework for graphics engines to use Render Graphs with explicit APIs.
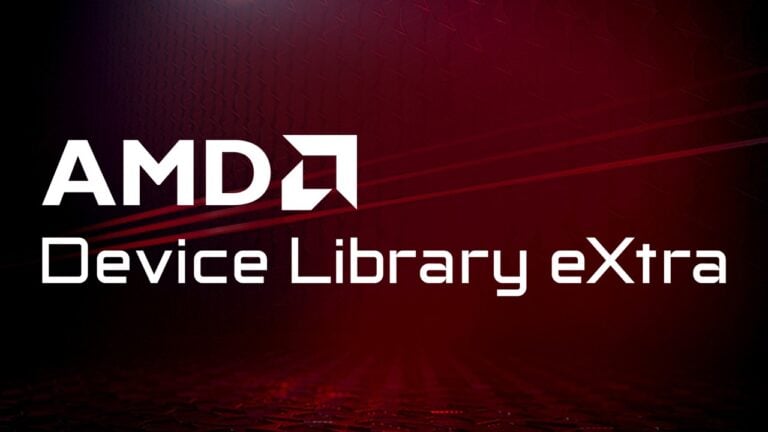
ADLX is a modern library designed to access features and functionality of AMD systems such as Display, 3D graphics, Performance Monitoring, GPU Tuning, and more.
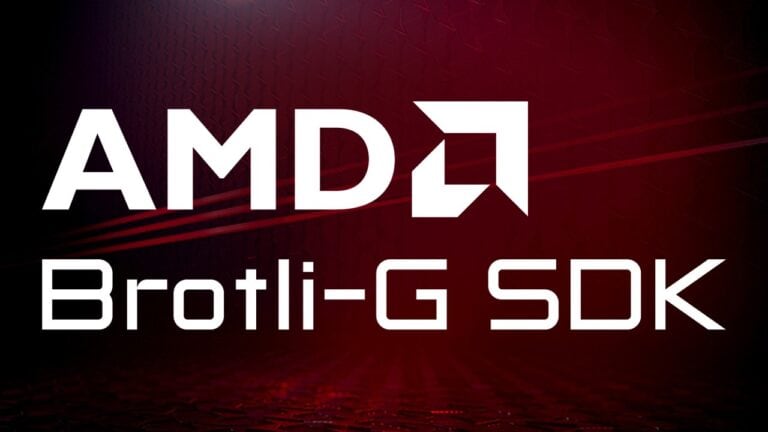
Brotli-G is an open-source compression/decompression standard for digital assets (based on Brotli) that is compatible with GPU hardware.
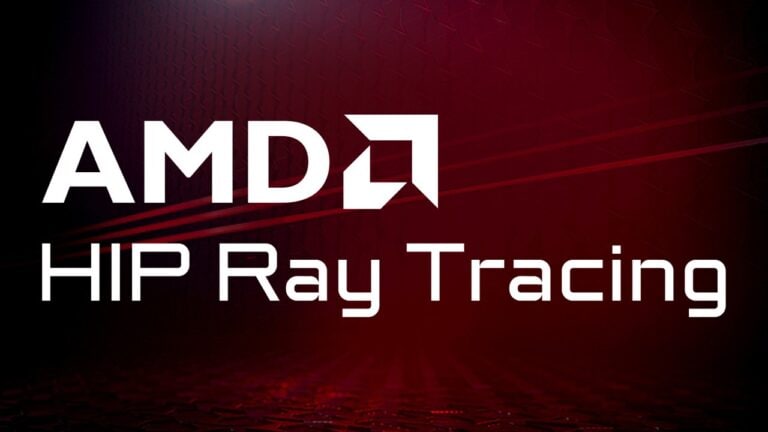
HIP RT is a ray tracing library for HIP, making it easy to write ray tracing applications in HIP.
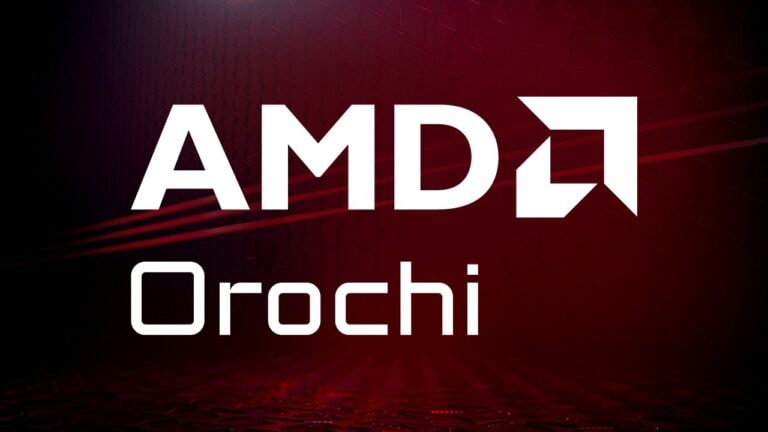
Orochi is a library which loads HIP and CUDA® APIs dynamically, allowing the user to switch APIs at runtime.
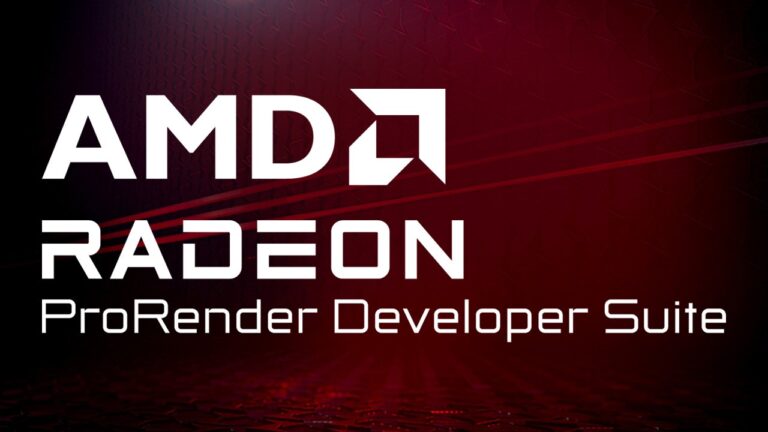
AMD Radeon™ ProRender is our fast, easy, and incredible physically-based rendering engine built on industry standards that enables accelerated rendering on virtually any GPU, any CPU, and any OS in over a dozen leading digital content creation and CAD applications.
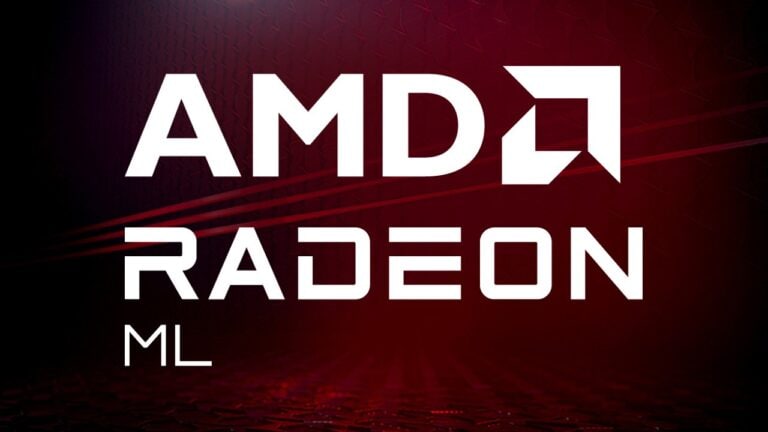
Radeon™ Machine Learning (Radeon™ ML or RML) is an AMD SDK for high-performance deep learning inference on GPUs.
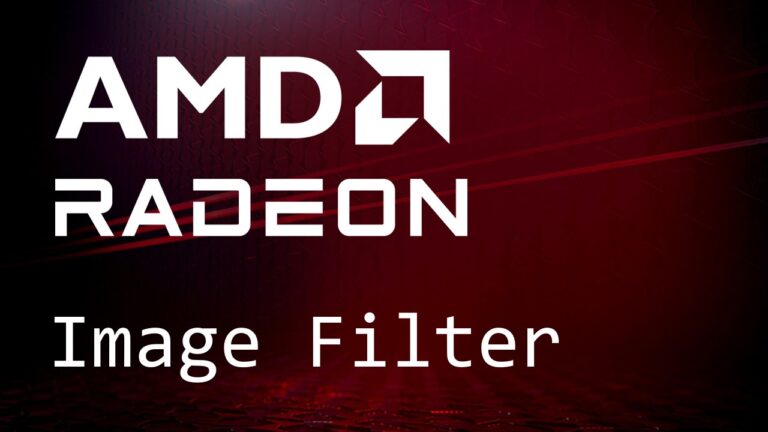
Harness the power of machine learning to enhance images with denoising, enabling your application to produce high quality images in a fraction of the time traditional denoising filters take.